Projects
3 Capstone Projects
Highest Package
22 LPA
Average Package
8 LPA
Who is this program for
BSC
Science graduates looking to transition into the IT industry.
BCA
Computer graduates aiming to become full-stack developers.
MCA
Postgraduates in computer who want to deepen their expertise.
B.Tech
Engineering graduates seeking training in full-stack development.
Learning journey at Stack Info Labs




Upskill Now
Live sessions, expert 1:1 doubt clearing, and quizzes.Capstone Projects
Work with industry experts on practical projects.Boost Profile
Mock interviews and resume-building sessionsCareer Goals
Get placement assistance with top companies.Explore our Syllabus
Curriculum is specifically engineered to meet the expectations of leading tech companies

L1 :- CS Fundamentals and DBMS
Topic 1: DBMS (Database Management Systems)
- Introduction to Databases
- The Relational Model
- Entity-Relationship Model
- Database Design
- Structured Query Language (SQL)
- Database Indexing and Optimization
- Transaction Management
- Database Security and Authorization
- Backup, Recovery, and Integrity
- Emerging Trends in Database Management
Topic 2: Networking Fundamentals
- Introduction to Computer Networking
- Network Topologies and Technologies
- OSI Model and Protocol Stack
- IP Addressing and Subnetting
- Routing and Switching
- Local Area Networks (LANs)
- Wide Area Networks (WANs)
- Wireless Networking
- Network Security
- Domain Name System (DNS)
- Cloud Networking
- Emerging Trends in Computer Networking
Topic 3: Operating System
- Introduction to Operating Systems
- Computer System Architecture
- Process Management
- Memory Management
- File System Management
- Input/Output (I/O) Systems
- User Interface and Command-Line Interaction
- System Security and Authentication
- Virtualization and Cloud Computing
- Distributed Operating Systems
- Real-Time Operating Systems (RTOS)

L2 :- Java Programming Fundamentals
Core Java Basics
- JDK
- Downloading, installation, and hello world development
- PATH environment Variable
- Local Variable Introduction
- Unary Operators: Increment (pre & post), Decrement (pre & post)
- Conditional Statements: if, else, switch
- Iterations: for, while, do-while loops
- Methods
- Global Variables
- Static Initialization Block (SIB)
- Multiple Classes
- Objects and Object-Oriented Principles
- Pass by Reference
- Constructors
- Instance Initialization Block (IIB)
- Encapsulation
- Inheritance
- Combining Constructor, this(), super(), SIB, IIB, Inheritance
- Packages and Access Levels
- Abstract Classes
- Interfaces
- Method Overloading and Overriding
- Usage of super and this in Methods
- Casting and Polymorphism
- Static Members and Polymorphism
- Keywords: final, this
- Enhanced for Loop
- Enums, Static Imports
- Inner Classes
- Annotations
- Wrapper Classes
Exception Handling
- Different Types of Abnormal Conditions
- Why Exception Handling is Required
- try, catch, finally Blocks
- Deviations to the finally Block
- Errors vs Exceptions
- Checked vs Unchecked Exceptions
- Common Error Types: StackOverflowError, OutOfMemoryError
- Common Exceptions: ArithmeticException, NullPointerException
- Custom Exceptions and User-Defined Exception Classes
Object Class
- toString()
- hashCode()
- equals()
- finalize()
- Garbage Collector
Reflection API
- clone()
- Deep Copy and Shallow Copy
- java.lang.Class Methods
- getFields(), getDeclaredFields()
- getMethods(), getDeclaredMethods()
Multi-Threading
- Multi-Tasking and Multi-Processing
- Types of Threads: User and Daemon
- Thread Class and Runnable Interface
- Thread Lifecycle
- Synchronization: Methods, Blocks, Deadlock
- Thread Communication: wait(), notify(), notifyAll()
- Thread Pool and Thread Group
Strings
- String Class and Methods: concat(), length(), charAt()
- StringBuilder vs StringBuffer
- String Memory Management
- Regular Expressions and Patterns
Arrays
- Declaration, Initialization, and Definition
- One-Dimensional and Multi-Dimensional Arrays
- Comparable and Comparator Interfaces
Collection API
- Limitations of Arrays
- List, Set, Queue, and Map Streams
- Important Classes: ArrayList, LinkedList, HashSet, HashMap
Generics
- Generic Types, Methods, and Wildcards
- Type Erasure and Restrictions
File Handling
- FileReader and FileWriter
- BufferedReader and BufferedWriter
- Serialization and Deserialization
Java 8 Features
- Lambda Expressions
- Functional Interfaces
- Stream API
- Optional Class
- Default and Static Methods in Interfaces
- Java Date and Time API
Servlets
- Introduction to Servlets
- Servlet Lifecycle
- Creating and Deploying Servlets
- ServletConfig and ServletContext
- Handling HTTP Requests and Responses
- Session Management in Servlets
- Cookies in Servlets
- Request Dispatcher and Redirects
- Filters in Servlets
- Annotations in Servlets
JSP (JavaServer Pages)
- Introduction to JSP
- JSP Lifecycle
- Directives in JSP
- JSP Scripting Elements: Declaration, Scriptlets, Expressions
- JSP Implicit Objects
- Standard Tag Library (JSTL)
- Custom Tags in JSP
- Exception Handling in JSP
- Using JavaBeans in JSP
- Session Management in JSP

L3 :- Data Structures and Algorithm
Basics of DSA
- Introduction to DSA
- Time and Space Complexity
- Big O Notation
- Common Asymptotic Notations
- Recursion and its Applications
- Divide and Conquer Approach
- Backtracking
Data Structures
- Arrays
- One-Dimensional Arrays
- Two-Dimensional Arrays
- Matrix Operations
- Strings
- Pattern Matching Algorithms
- KMP Algorithm
- Z Algorithm
- Rabin-Karp
- Linked Lists
- Singly Linked List
- Doubly Linked List
- Circular Linked List
- Stacks
- Stack Implementation
- Applications of Stacks
- Queues
- Queue Implementation
- Circular Queue
- Deque (Double-Ended Queue)
- Trees
- Binary Trees
- Binary Search Tree (BST)
- AVL Trees
- Segment Trees
- Fenwick Tree (Binary Indexed Tree)
- Heaps
- Min-Heap
- Max-Heap
- Heap Sort
- Hashing
- Hash Tables
- Collision Resolution Techniques
- Tries
- Disjoint Set Union (DSU)
Searching and Sorting
- Linear Search
- Binary Search
- Ternary Search
- Bubble Sort
- Insertion Sort
- Selection Sort
- Merge Sort
- Quick Sort
- Heap Sort
- Counting Sort
- Radix Sort
- Bucket Sort
Graphs
- Graph Representation
- Adjacency Matrix
- Adjacency List
- Graph Traversal
- Breadth-First Search (BFS)
- Depth-First Search (DFS)
- Shortest Path Algorithms
- Dijkstra's Algorithm
- Bellman-Ford Algorithm
- Floyd-Warshall Algorithm
- Minimum Spanning Tree (MST)
- Kruskal's Algorithm
- Prim's Algorithm
- Cycle Detection
- Topological Sorting
- Graph Coloring
- Strongly Connected Components (SCC)
- Articulation Points and Bridges
Dynamic Programming (DP)
- Introduction to DP
- Memoization and Tabulation
- 1D DP Problems
- Fibonacci Sequence
- Climbing Stairs
- House Robber Problem
- 2D DP Problems
- Knapsack Problem
- Longest Common Subsequence (LCS)
- Longest Increasing Subsequence (LIS)
- Advanced DP Problems
- Matrix Chain Multiplication
- Word Break Problem
- Subset Sum Problem
Advanced DSA Topics
- Bit Manipulation
- Sliding Window Technique
- Two Pointer Technique
- Greedy Algorithms
- Divide and Conquer
- Segment Trees and Lazy Propagation
- Fenwick Tree (Binary Indexed Tree)

L4 :- Spring Boot and Web Services
Topic 1: Spring Boot
- Introduction to Spring Boot
- Setting up a Spring Boot Project
- Spring Boot Annotations
- Configuring Spring Boot Properties
- Creating REST APIs
- Spring Boot DevTools
- Spring Boot Starter Modules
- Spring Boot Actuator
- Spring Data JPA
- Spring Security
- Spring Boot Testing
- External Configuration (application.yml, application.properties)
- Development and Deployment: JAR vs WAR
- Spring Boot Profiles
Topic 2: Web Services
- Introduction to Web Services
- SOAP vs REST
- Creating RESTful Web Services in Spring Boot
- Media Types and Content Negotiation
- Consuming REST APIs from a Spring Boot Application
- Spring MVC vs Spring Boot
- HTTP Methods: GET, POST, PUT, DELETE
- Exception Handling in REST Services
- Authentication and Authorization in Web Services
- Spring Cloud and Spring Boot Microservices
- Swagger for API Documentation
- Testing Web Services with Postman

L4 :- System Design
Topic 1: Introduction to System Design
- What is System Design?
- Goals of System Design
- Components of a System Design
- System Design Process
- Importance of System Design
- Types of Systems
- Architecture of a System
Topic 2: Design Principles
- SOLID Principles
- KISS Principle
- DRY Principle
- YAGNI Principle
Topic 3: Design Patterns
- Creational Patterns
- Singleton Pattern
- Factory Pattern
- Abstract Factory Pattern
- Builder Pattern
- Prototype Pattern
- Structural Patterns
- Adapter Pattern
- Bridge Pattern
- Composite Pattern
- Decorator Pattern
- Facade Pattern
- Flyweight Pattern
- Proxy Pattern
- Behavioral Patterns
- Observer Pattern
- Strategy Pattern
- Command Pattern
- State Pattern
- Template Method Pattern
- Chain of Responsibility Pattern
- Interpreter Pattern
- Iterator Pattern
- Mediator Pattern
- Observer Pattern
- Visitor Pattern
Topic 4: Low-Level Design (LLD)
- Understanding the Purpose and Scope of LLD
- LLD Design Process
- Types of LLD
- Creating a Low-Level Design Document
Topic 5: High-Level Design (HLD)
- Understanding the Purpose and Scope of HLD
- HLD Design Process
- Types of HLD
- Creating a High-Level Design Document
Topic 6: Scalability and Performance
- Scalability
- Performance
- Load Balancing
- Caching
- Database Scaling
Topic 7: Security and Reliability
- Security
- Reliability
- Fault Tolerance
- Disaster Recovery
- Backup and Restore
Topic 8: Integration and Deployment
- Continuous Integration
- Continuous Deployment
- DevOps
- Microservices

L6 :- Project Development / Testing / Deployment
Topic 1: Building a Spring Boot Application
- Creating a new Spring Boot project
- Dependency management with Maven or Gradle
- Defining application properties (e.g., database configuration, logging)
Topic 2: Working with Databases
- Integrating Spring Boot with relational databases (e.g., MySQL, PostgreSQL)
- Using Spring Data JPA for database interactions
- Implementing CRUD operations with JPA repositories
Topic 3: Securing a Spring Boot Application
- Implementing authentication and authorization
- Securing REST APIs with Spring Security
- Handling CSRF attacks and other security considerations
Topic 4: Building and Packaging Spring Boot Applications
- Creating executable JARs or WARs
- Deploying Spring Boot applications to local and remote servers
Topic 5: Developing a Basic Spring Boot Application
- Creating and configuring Spring Beans
- Implementing RESTful APIs with Spring Web
- Handling HTTP requests and responses
- Data validation and error handling
Topic 6: Testing Spring Boot Applications
- Writing unit tests for Spring components (e.g., controllers, services)
- Using JUnit and Mockito for testing
- Understanding test best practices and test coverage
Topic 7: Spring Boot Actuator
- Monitoring and managing Spring Boot applications using Actuator endpoints
- Customizing Actuator endpoints and security configurations
Topic 8: Containerization and Deployment
- Introduction to Docker and containerization
- Dockerizing a Spring Boot application
- Deploying Spring Boot apps to cloud platforms (e.g., AWS, Azure, Google Cloud)
Topic 9: Containerization and Deployment
- Setting up CI/CD pipelines with tools like Jenkins or GitLab CI
- Automating the deployment process
Topic 10: Monitoring and Scaling
- Utilizing monitoring tools (e.g., Spring Boot Admin, Prometheus, Grafana)
- Scaling Spring Boot applications in different deployment environments
Topic 11: Troubleshooting and Best Practices
- Identifying and resolving common issues
- Adopting best practices for Spring Boot application development and deployment
Get job ready within 6 months with Career Services PRO

Placement Support

Mock Interviews

Resume BuildUp

Unlimited Interview Calls
Unleash your career potential with unlimited job access, interview support, and profile review.
Receive unlimited interview calls from a diverse pool of interested employers/recruiters until you successfully secure a job.
Find the best suited job role that meets your career and salary expectations.

Dedicated Placement Cell
Job roles & companies you can target
Software Developer
Systems Architech
Web Developer
Front-end Developer
Product Manager
Software Tester
Quality Assurance
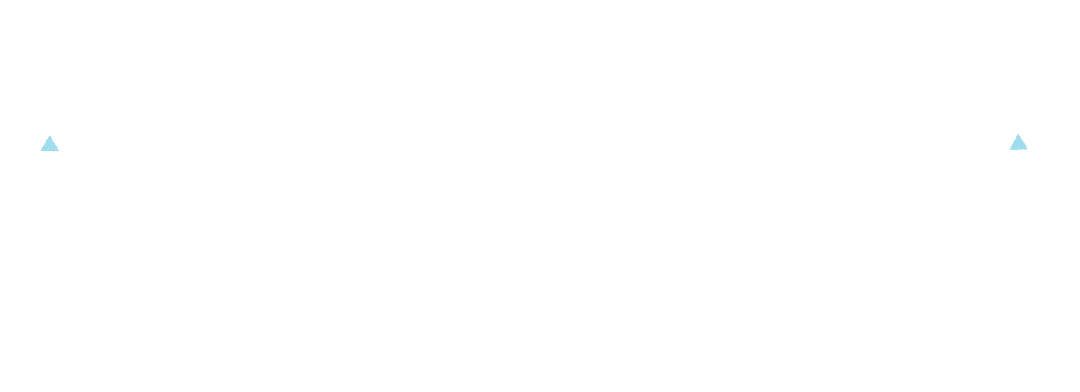
Fee & Batch Details
Scholarship Details

Scholarships are awarded based on profile review. Eligible candidates can avail upto 20% scholarship on desired program. Click the button below to apply.
Program Fee
₹ 30,000 + 18% GST
EMI Options
Pay in two EMIs
₹ 15,000/month
Batch Details